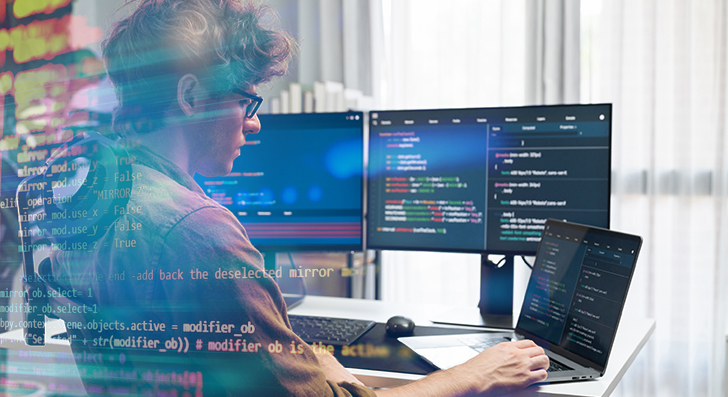
Scalability implies your software can cope with progress—much more users, additional knowledge, and even more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and practical guidebook that will help you begin by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it ought to be aspect of one's approach from the beginning. Lots of apps fail if they develop rapid mainly because the original structure can’t manage the additional load. Like a developer, you need to Assume early about how your technique will behave under pressure.
Begin by building your architecture for being adaptable. Steer clear of monolithic codebases where by every little thing is tightly related. Rather, use modular layout or microservices. These patterns split your application into smaller, impartial sections. Each module or support can scale By itself without having affecting The complete technique.
Also, consider your database from day just one. Will it have to have to handle a million end users or simply a hundred? Choose the appropriate form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only will work less than present-day conditions. Think about what would come about If the person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that support scaling, like message queues or party-driven methods. These assist your app tackle extra requests without the need of having overloaded.
Any time you Make with scalability in mind, you are not just getting ready for success—you're lessening long run complications. A effectively-planned system is less complicated to keep up, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the appropriate Databases
Choosing the right databases is actually a important A part of developing scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down as well as result in failures as your application grows.
Get started by knowledge your info. Can it be hugely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely potent with associations, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to manage much more website traffic and information.
In the event your facts is more versatile—like person action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured information and will scale horizontally much more simply.
Also, consider your go through and generate patterns. Do you think you're accomplishing a lot of reads with less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases which can deal with substantial produce throughput, or even occasion-based mostly data storage techniques like Apache Kafka (for short term facts streams).
It’s also good to Believe ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data depending on your access patterns. And constantly watch databases effectiveness when you improve.
To put it briefly, the appropriate databases will depend on your application’s framework, pace desires, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll conserve lots of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your application grows, every single tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Make productive logic from the beginning.
Commence by creating clean up, uncomplicated code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most complex Alternative if an easy 1 operates. Keep your features brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take much too prolonged to run or uses an excessive amount memory.
Up coming, evaluate your database queries. These normally sluggish matters down a lot more than the code alone. Be sure each query only asks for the data you truly need. Prevent Choose *, which fetches anything, and rather decide on specific fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily throughout large tables.
Should you detect exactly the same knowledge remaining requested over and over, use caching. Retail outlet the results temporarily working with applications like Redis or Memcached so that you don’t have to repeat pricey operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with significant datasets. Code and queries that function fantastic with one hundred documents might crash once they have to deal with 1 million.
In a nutshell, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when required. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more targeted traffic. If anything goes through one server, it will quickly become a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app quick, stable, and scalable.
Load balancing spreads incoming targeted visitors throughout multiple servers. Instead of one server accomplishing many of the get the job done, the load balancer routes end users to distinct servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Other folks. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused promptly. When consumers ask for the exact same details again—like an item web page or a profile—you don’t need to fetch it with the database when. It is possible to serve it with the cache.
There are two popular forms of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for quick entry.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And constantly be sure your cache is updated when knowledge does improve.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your application tackle much more end users, continue to be quick, and Get well from complications. If you intend to improve, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you may need applications that let your app expand simply. That’s where by cloud platforms and containers come in. They give you versatility, lessen setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Expert services (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and services as you'll need them. You don’t must acquire hardware or guess foreseeable future ability. When website traffic boosts, you could increase extra sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your application in lieu of taking care of infrastructure.
Containers are A different essential Software. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for performance and trustworthiness.
In brief, working with cloud and container resources usually means you'll be able to scale fast, deploy simply, and recover speedily when problems come about. If you want your application to mature without having restrictions, begin working with these tools early. They help save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Watch Every thing
In case you don’t observe your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved choices as your application grows. It’s a vital part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your check here servers—observe your application much too. Keep an eye on how much time it will take for end users to load web pages, how often problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes previously mentioned a limit or perhaps a service goes down, you should get notified immediately. This allows you take care of difficulties fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, targeted traffic and information maximize. With no monitoring, you’ll pass up indications of difficulties till it’s much too late. But with the best tools set up, you remain on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for significant organizations. Even compact apps have to have a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you can Create applications that expand efficiently without breaking under pressure. Start out little, Consider significant, and Develop clever.